- Published on
Unleashing the Full Potential of BigQuery for Advanced Marketing Campaign Analysis
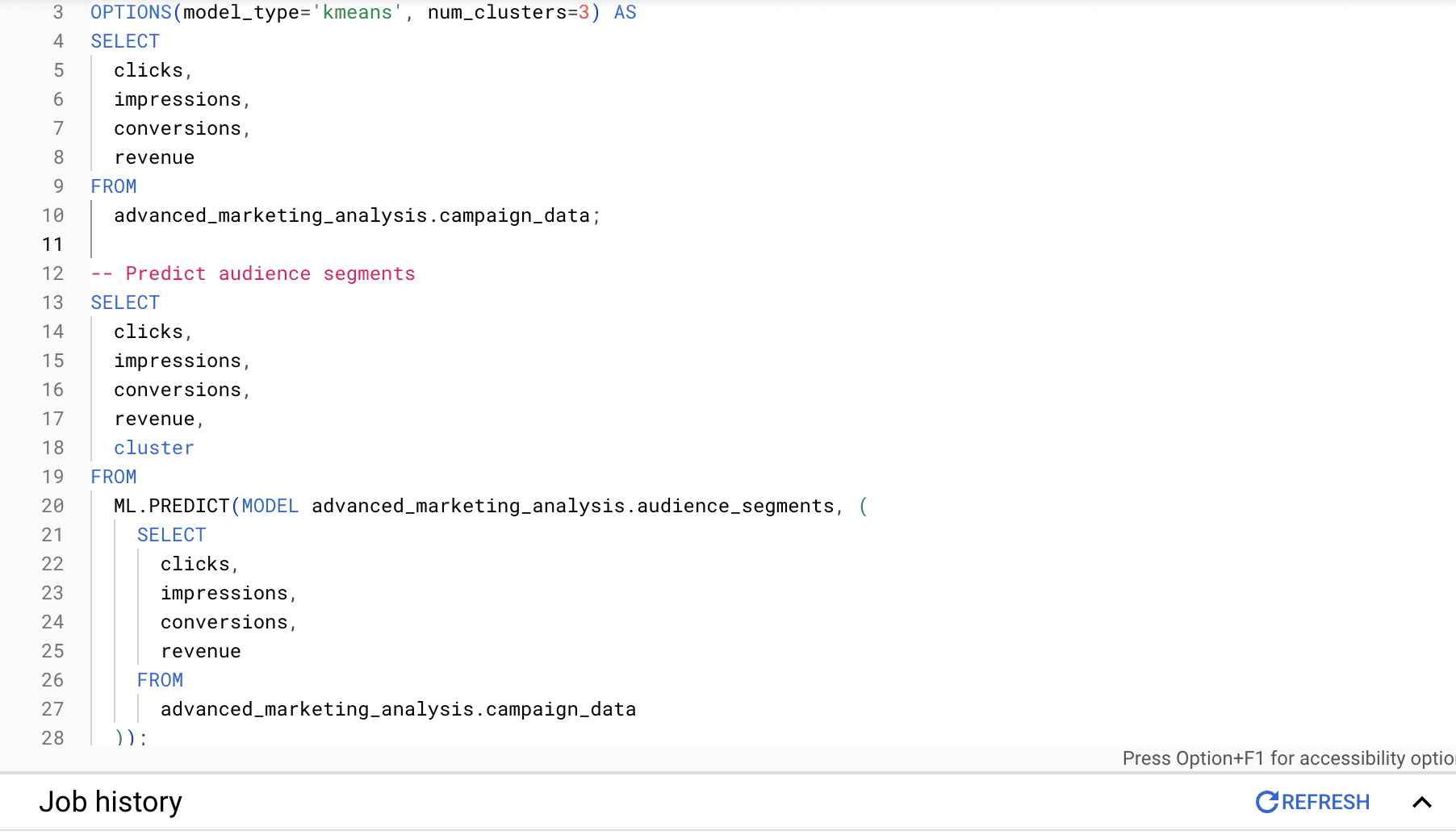
Introduction: Transforming Marketing Analytics with BigQuery
In today’s competitive digital landscape, marketing analytics has evolved from simple data collection to sophisticated data science. Google BigQuery stands at the forefront of this transformation, enabling businesses to perform complex, high-level analyses on massive datasets. This blog post dives deep into advanced marketing campaign analysis using BigQuery, illustrated with a real-world case study. We’ll explore detailed techniques, code snippets, and expert tips to help you maximize your marketing insights and drive strategic decisions.
Setting Up BigQuery for Advanced Analysis
Provisioning BigQuery and Data Sources
Begin by ensuring your BigQuery environment is properly set up. This involves provisioning the necessary datasets and tables, and importing your marketing data from various sources such as Google Analytics, CRM systems, and third-party ad platforms.
-- Create datasets for advanced analysis
CREATE SCHEMA IF NOT EXISTS advanced_marketing_analysis;
-- Create table for campaign data
CREATE TABLE IF NOT EXISTS advanced_marketing_analysis.campaign_data (
campaign_id STRING,
campaign_name STRING,
start_date DATE,
end_date DATE,
clicks INT64,
impressions INT64,
cost FLOAT64,
conversions INT64,
revenue FLOAT64
);
Automating Data Ingestion
Automate data ingestion using Cloud Functions and Cloud Scheduler to keep your data up-to-date without manual intervention.
# Python script for Cloud Function to load data into BigQuery
from google.cloud import bigquery
import pandas as pd
def load_data_to_bigquery(event, context):
client = bigquery.Client()
table_id = 'your-project.advanced_marketing_analysis.campaign_data'
dataframe = pd.read_csv('gs://your-bucket/campaign_data.csv')
job = client.load_table_from_dataframe(dataframe, table_id)
job.result()
Advanced Data Transformation Techniques
Leveraging SQL for Complex Transformations
Utilize BigQuery’s SQL capabilities to perform complex data transformations. This includes window functions, CTEs, and advanced aggregations.
-- Calculate running totals and moving averages for campaign performance
WITH campaign_stats AS (
SELECT
campaign_id,
campaign_name,
DATE(start_date) AS date,
clicks,
impressions,
cost,
conversions,
revenue
FROM
advanced_marketing_analysis.campaign_data
)
SELECT
campaign_id,
campaign_name,
date,
SUM(clicks) OVER (PARTITION BY campaign_id ORDER BY date) AS running_total_clicks,
AVG(clicks) OVER (PARTITION BY campaign_id ORDER BY date ROWS BETWEEN 6 PRECEDING AND CURRENT ROW) AS moving_avg_clicks,
SUM(revenue) OVER (PARTITION BY campaign_id ORDER BY date) AS running_total_revenue
FROM
campaign_stats
ORDER BY
campaign_id, date;
Data Enrichment with Third-Party APIs
Enhance your campaign data by integrating third-party APIs, such as weather data or social media sentiment, to add contextual insights.
# Example Python script to enrich data with weather API
import requests
from google.cloud import bigquery
def enrich_with_weather_data(event, context):
client = bigquery.Client()
query = """
SELECT DISTINCT DATE(start_date) AS date
FROM advanced_marketing_analysis.campaign_data
WHERE weather IS NULL
"""
dates = [row['date'] for row in client.query(query)]
for date in dates:
response = requests.get(f'https://api.weather.com/v1/date/{date}?apikey=YOUR_API_KEY')
weather_data = response.json()
update_query = f"""
UPDATE advanced_marketing_analysis.campaign_data
SET weather = '{weather_data['weather_description']}'
WHERE DATE(start_date) = '{date}'
"""
client.query(update_query)
Predictive Analytics: Forecasting Future Trends
Building Predictive Models with BigQuery ML
Leverage BigQuery ML to create and deploy machine learning models directly within BigQuery. We’ll build a model to predict future conversions based on historical data.
-- Create a linear regression model to predict conversions
CREATE OR REPLACE MODEL advanced_marketing_analysis.conversion_forecast
OPTIONS(model_type='linear_reg') AS
SELECT
clicks,
impressions,
cost,
revenue,
conversions
FROM
advanced_marketing_analysis.campaign_data;
-- Evaluate the model
SELECT
*
FROM
ML.EVALUATE(MODEL advanced_marketing_analysis.conversion_forecast, (
SELECT
clicks,
impressions,
cost,
revenue,
conversions
FROM
advanced_marketing_analysis.campaign_data
));
Applying Machine Learning Predictions
Use the trained model to predict future conversions and optimize your campaign strategies.
-- Predict future conversions
SELECT
clicks,
impressions,
cost,
revenue,
predicted_conversions
FROM
ML.PREDICT(MODEL advanced_marketing_analysis.conversion_forecast, (
SELECT
clicks,
impressions,
cost,
revenue
FROM
advanced_marketing_analysis.campaign_data
));
Advanced Audience Segmentation
Behavioral Segmentation Using Clustering
Implement clustering algorithms to segment your audience based on behavior and engagement metrics.
-- Create a K-means clustering model for audience segmentation
CREATE OR REPLACE MODEL advanced_marketing_analysis.audience_segments
OPTIONS(model_type='kmeans', num_clusters=3) AS
SELECT
clicks,
impressions,
conversions,
revenue
FROM
advanced_marketing_analysis.campaign_data;
-- Predict audience segments
SELECT
clicks,
impressions,
conversions,
revenue,
cluster
FROM
ML.PREDICT(MODEL advanced_marketing_analysis.audience_segments, (
SELECT
clicks,
impressions,
conversions,
revenue
FROM
advanced_marketing_analysis.campaign_data
));
Personalizing Campaigns Based on Segments
With audience segments identified, tailor your marketing messages and strategies to each segment for increased engagement and conversions.
Time Series Analysis for Campaign Performance
Decomposing Time Series Data
Perform time series decomposition to identify trends, seasonality, and noise in your campaign performance data.
-- Decompose time series data for campaign clicks
WITH time_series_data AS (
SELECT
DATE(start_date) AS date,
SUM(clicks) AS total_clicks
FROM
advanced_marketing_analysis.campaign_data
GROUP BY
date
)
SELECT
date,
total_clicks,
EXTRACT(YEAR FROM date) AS year,
EXTRACT(MONTH FROM date) AS month,
EXTRACT(DAY FROM date) AS day
FROM
time_series_data
ORDER BY
date;
Forecasting with Time Series Models
Use time series forecasting models to predict future campaign performance and optimize resource allocation.
-- Create a time series model to forecast future clicks
CREATE OR REPLACE MODEL advanced_marketing_analysis.click_forecast
OPTIONS(model_type='arima') AS
SELECT
date,
total_clicks
FROM
time_series_data;
-- Forecast future clicks
SELECT
forecast_timestamp,
predicted_clicks,
prediction_interval_lower_bound,
prediction_interval_upper_bound
FROM
ML.FORECAST(MODEL advanced_marketing_analysis.click_forecast, STRUCT(30 AS horizon));
Attribution Modeling: Understanding Campaign Impact
Multi-Touch Attribution Analysis
Implement multi-touch attribution models to understand the contribution of each touchpoint in the customer journey.
-- Create a multi-touch attribution model
WITH touchpoints AS (
SELECT
user_id,
campaign_id,
campaign_name,
event_time,
event_type,
revenue
FROM
advanced_marketing_analysis.user_journey
)
SELECT
user_id,
campaign_id,
campaign_name,
event_time,
event_type,
revenue,
SUM(revenue) OVER (PARTITION BY user_id ORDER BY event_time) AS cumulative_revenue
FROM
touchpoints
ORDER BY
user_id, event_time;
Visualizing Attribution Paths
Use visualization tools to create Sankey diagrams or path analysis charts that illustrate the customer journey and attribution paths.
Optimizing Campaigns with A/B Testing
Designing and Analyzing A/B Tests
Set up A/B tests to compare different campaign variations and identify the most effective strategies.
-- Example A/B test setup
CREATE TABLE IF NOT EXISTS advanced_marketing_analysis.ab_test_results (
test_id STRING,
variant STRING,
impressions INT64,
clicks INT64,
conversions INT64,
revenue FLOAT64
);
-- Analyze A/B test results
SELECT
test_id,
variant,
(SUM(clicks) / SUM(impressions)) * 100 AS ctr,
(SUM(conversions) / SUM(clicks)) * 100 AS conversion_rate,
AVG(revenue) AS avg_revenue
FROM
advanced_marketing_analysis.ab_test_results
GROUP BY
test_id, variant
ORDER BY
test_id, variant;
Interpreting Test Results
Draw actionable insights from A/B test results to refine your marketing strategies and improve overall campaign performance.
Business Impact: Driving Strategic Decisions with Data
Enhancing Decision-Making with Advanced Analytics
Harness the power of BigQuery to drive strategic decisions that align with your business goals. Advanced analytics provide deeper insights into campaign performance, customer behavior, and market trends.