- Published on
Mastering the Art of IFrames with Selenium
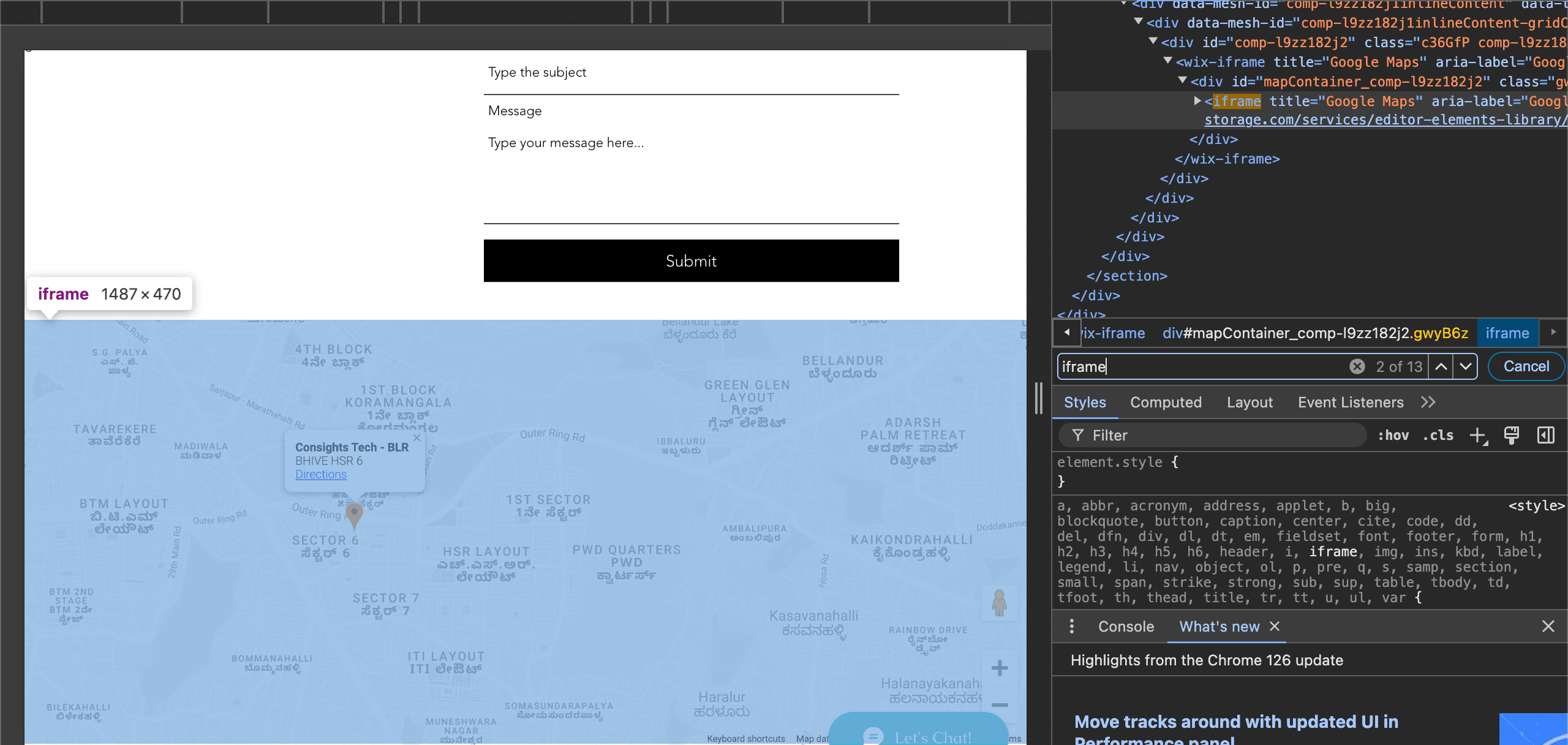
IFrames (Inline Frames) are a powerful tool for embedding external content within web pages. While they offer benefits like modularity and dynamic content loading, they present unique challenges for web automation, particularly when using Selenium. This article provides a comprehensive guide to navigating and interacting with iframes using Selenium, equipping you with the knowledge and techniques to overcome these challenges.
Understanding IFrames and Their Significance
IFrames essentially embed a separate HTML document within the current one, creating a "mini-browser" inside your main browser window. This allows seamless integration of content from different sources without altering the parent page's structure. This is invaluable for embedding interactive elements, advertisements, maps, and other external applications.
However, this separation introduces complexities for web automation tools like Selenium. Each iframe operates within its own browsing context, making it crucial to explicitly switch Selenium's focus to the correct iframe before interacting with its elements.
Selenium and the Art of IFrame Navigation
Selenium provides dedicated methods to handle iframes. The core concept is switching the driver's context to target the desired frame. Let's explore the techniques involved.
Switching to an IFrame
Switching to an iframe is essential for interacting with elements contained within it. This can be achieved using several methods:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = webdriver.Chrome()
driver.get("http://example.com") # Replace with a page containing iframes
# 1. Switching by ID or Name:
driver.switch_to.frame("iframe_id_or_name")
# 2. Switching by Web Element:
iframe_element = driver.find_element(By.XPATH, "//iframe[@src='...']") # Locate the iframe element
driver.switch_to.frame(iframe_element)
# 3. Switching by Index: (Less reliable, use with caution)
driver.switch_to.frame(0) # Switches to the first iframe on the page
Returning to the Parent Frame
After interacting with the iframe's contents, you'll likely need to return to the main page's context. This is accomplished with:
driver.switch_to.default_content() # Returns to the top-level browsing context
Handling Multiple IFrames
When dealing with multiple iframes, you need to switch between them appropriately.
# Switch to the first iframe
driver.switch_to.frame("first_iframe")
# Perform actions in the first iframe
first_element = driver.find_element_by_id("first_element_id")
first_element.click()
# Switch back to the main document
driver.switch_to.default_content()
# Switch to the second iframe
driver.switch_to.frame("second_iframe")
# Perform actions in the second iframe
second_element = driver.find_element_by_id("second_element_id")
second_element.click()
# Switch back to the main document
driver.switch_to.default_content()
Handling Nested IFrames
Nested iframes, or iframes within iframes, add another layer of complexity. Navigating them requires switching contexts sequentially:
# Switch to the first level iframe
driver.switch_to.frame("first_level_iframe")
# Switch to the nested iframe within the first level iframe
driver.switch_to.frame("nested_iframe")
# Perform actions within the nested iframe
nested_element = driver.find_element_by_id("nested_element_id")
nested_element.click()
# Switch back to the first level iframe
driver.switch_to.parent_frame()
# Switch back to the main document
driver.switch_to.default_content()
Understanding and managing nested iframes is critical for complex web applications where content is deeply nested, ensuring that all elements are accessible for automation.
Addressing Common Challenges
Working with iframes can present challenges, especially regarding timing and element visibility.
Synchronization Issues and Explicit Waits
IFrames might load asynchronously. Trying to interact with elements before the iframe has fully loaded will result in errors. Explicit waits are crucial:
wait = WebDriverWait(driver, 10)
iframe = wait.until(EC.frame_to_be_available_and_switch_to_it((By.ID, "iframe_id")))
# Now interact with elements within the iframe
Element Visibility
Ensure elements are visible and interactable before attempting actions. Combine explicit waits with visibility checks:
element = wait.until(EC.visibility_of_element_located((By.ID, "element_inside_iframe")))
element.click()
Best Practices for IFrame Handling
- Prioritize Explicit Waits: Always use explicit waits to ensure elements are loaded and ready.
- Modularize Your Code: Create reusable functions for switching to and interacting within iframes.
- Validate IFrame Presence: Check if the iframe exists before attempting to switch to it.
- Leverage Selenium's Capabilities: Utilize WebDriverWait, ExpectedConditions, and other Selenium features for robust automation.
Conclusion
IFrames, while powerful, require careful handling in web automation. By understanding the context switching mechanisms, utilizing explicit waits, and following best practices, you can effectively tame iframes with Selenium. This enables comprehensive testing and interaction with even the most complex web applications, ensuring a smoother and more reliable automation experience.