- Published on
Automate Data Entry with Google Sheets, Apps Script and API
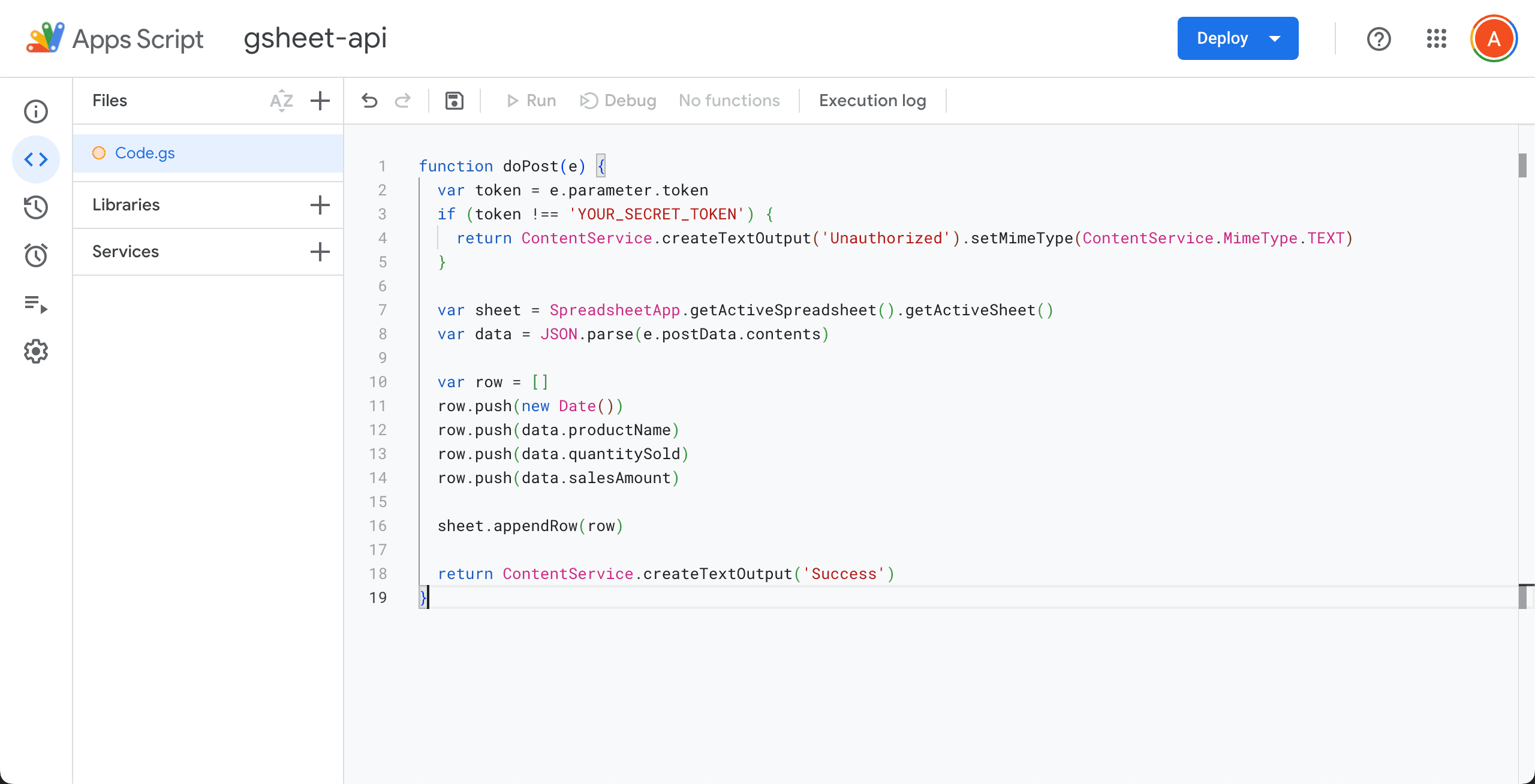
Automate Data Entry with Google Sheets, Apps Script, and an API
This article shows you how to use Google Apps Script to create an API that automatically adds data to your Google Sheets. No more manual entry! This saves time, reduces errors, and boosts productivity.
Getting Started with Google Apps Script
Google Apps Script is a cloud-based platform that lets you write small applications that work within Google's ecosystem. Since it's based on JavaScript, it's pretty easy to pick up if you have some coding experience. We'll use it to build an API that talks to your Google Sheets.
Automating data entry frees up your team to focus on more important work. This API integration ensures your data is always up-to-date and improves how everyone works together.
Preparing Your Google Sheet
First, create a new Google Sheet where your data will live. Make sure the sheet has clear headers. For example, if you're tracking sales, your headers might be "Date," "Product Name," "Quantity Sold," and "Sales Amount." Well-organized headers will help you map incoming data to the right columns and make your data easier to read and analyze.

Opening the Apps Script Editor
To write the script, open your Google Sheet and go to "Extensions > Apps Script." This opens the Apps Script editor. It's a straightforward interface where you'll write the code that receives data and adds it to your sheet.
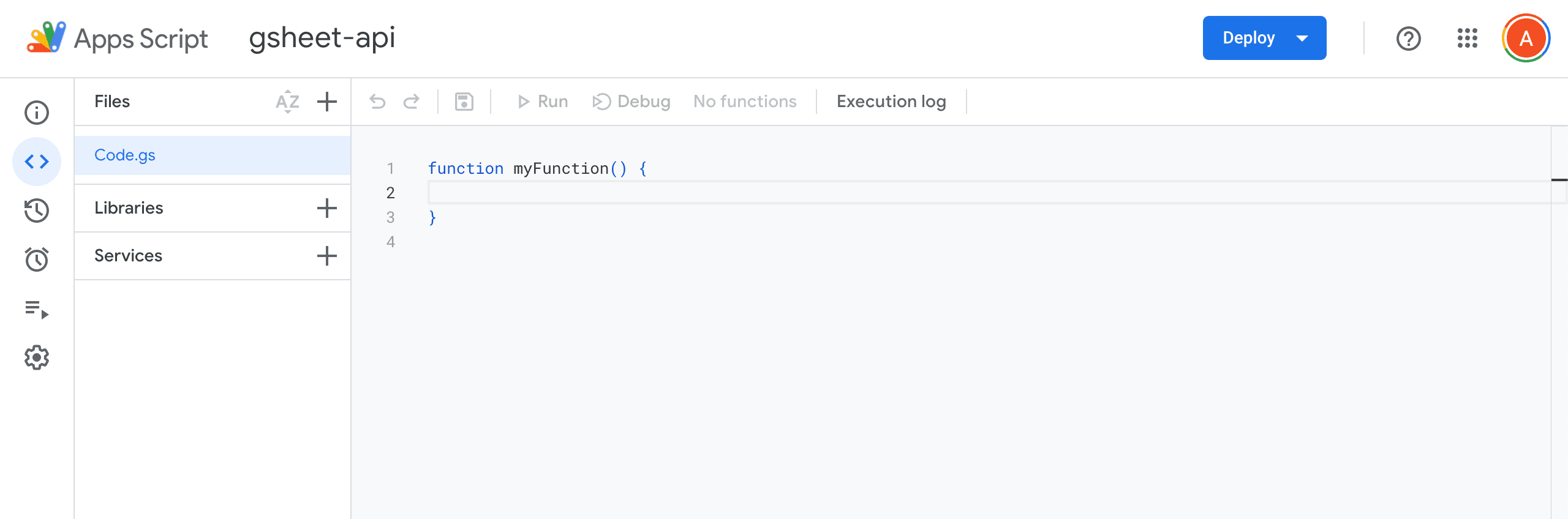
Writing the Data-Receiving Function
Here’s the core function that receives and processes the data:
function doPost(e) {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var data = JSON.parse(e.postData.contents);
sheet.appendRow([
new Date(), // Assuming the first column is Date
data.productName,
data.quantitySold,
data.salesAmount
]);
return ContentService.createTextOutput('Success');
}
This doPost
function handles incoming POST requests. It takes the data, formats it into a row, and adds it to your sheet.
Deploying as a Web App
Now, deploy your script as a web app. This creates a URL that you'll use to send data to your sheet. In the Apps Script editor, click "Deploy > New deployment." Choose "Web app," set "Execute as" to "Me," and "Who has access" to "Anyone" (we'll address security shortly). Deploying generates a unique URL – keep this secure, as it gives access to your sheet.
Testing Your API
Before connecting your API to other applications, test it using a tool like Postman. Send POST requests to your web app URL with sample data like this:
{
"productName": "Example Product",
"quantitySold": 10,
"salesAmount": 150.0
}

Test with different data to ensure everything works as expected.
Securing Your API
It's crucial to secure your API. A simple method is using an authentication token. Add a token check to your doPost
function:
function doPost(e) {
var token = e.parameter.token
if (token !== 'YOUR_SECRET_TOKEN') {
return ContentService.createTextOutput('Unauthorized').setMimeType(ContentService.MimeType.TEXT)
}
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet()
var data = JSON.parse(e.postData.contents)
var row = []
row.push(new Date())
row.push(data.productName)
row.push(data.quantitySold)
row.push(data.salesAmount)
sheet.appendRow(row)
return ContentService.createTextOutput('Success')
}
Now, only requests with the correct token can add data to your sheet. Remember to store this token securely and never expose it in your code repository. Consider using Properties Service to store the token.
Integrating with Other Applications
With your API tested and secured, you can connect it to other apps. Whether it's a CRM, e-commerce platform, or custom software, you can configure them to send data directly to your sheet via the API. Tools like Zapier can help automate this process, triggering data transfer based on specific events.
Monitoring and Maintenance
Regularly check your data and script for any issues. Use the Apps Script dashboard to view execution logs. Consider setting up email error notifications or using Google Cloud Logging for more advanced monitoring.
Conclusion
Manual data entry is a thing of the past. By harnessing the combined power of Google Apps Script and APIs, you can transform your workflows, boost productivity, and empower your team to focus on what matters most. Happy Automating!