- Published on
Beyond the Basics - Advanced Google Apps Script Techniques for Data Automation
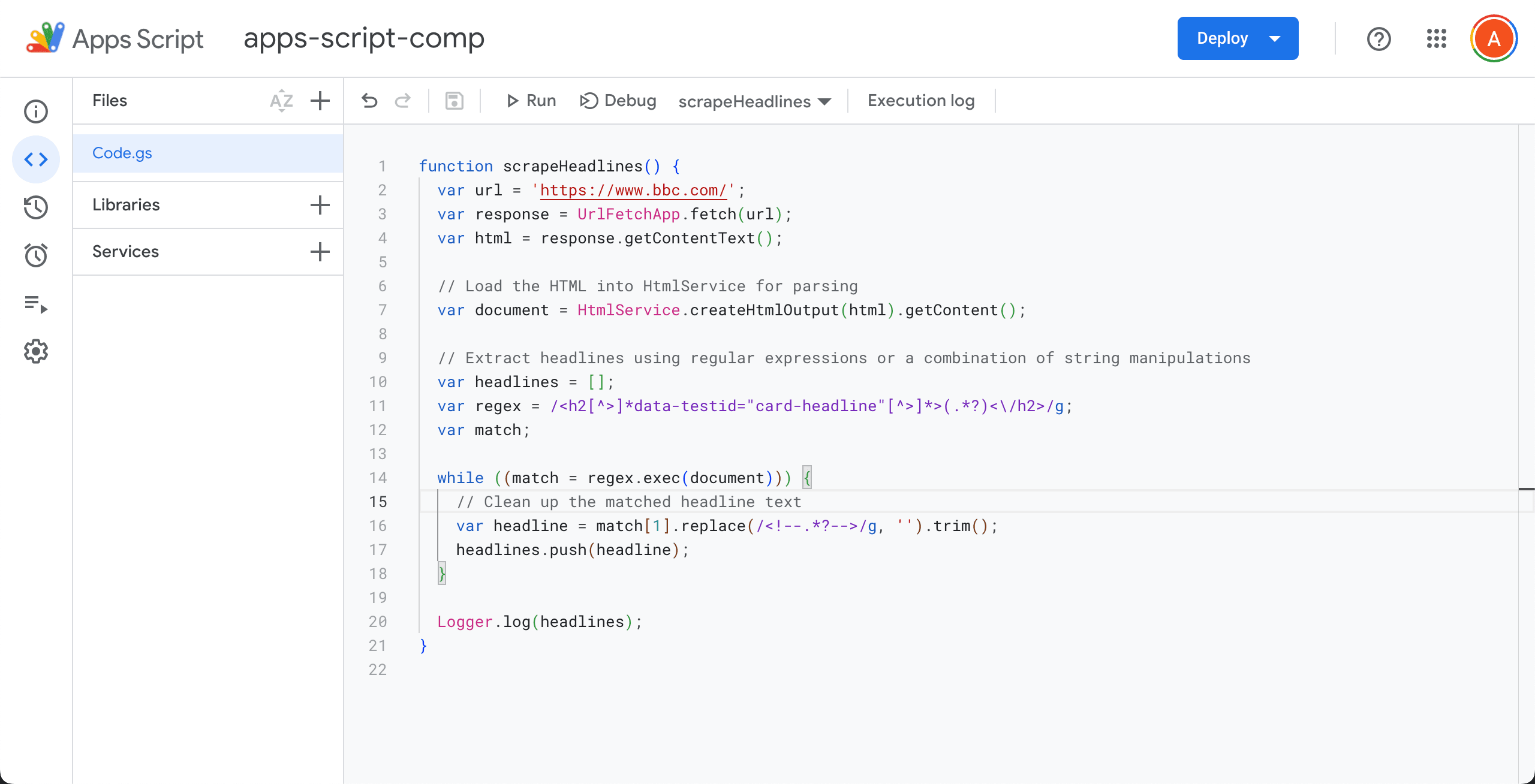
Introduction: Taming Tedious Tasks with Automation
Ever find yourself bogged down by repetitive data tasks? I know I have. Imagine effortlessly extracting data from websites, automatically generating reports, and sending timely email notifications – all within your familiar Google Workspace environment. This guide unlocks the potential of Google Apps Script, providing practical examples and empowering you to automate data-driven tasks.
Identifying Automation Opportunities
The first step is figuring out which tasks are good candidates for automation. Look for tasks that are:
- Repetitive: Do you find yourself doing the same thing over and over?
- Time-consuming: Does the task take up a significant chunk of your day?
- Prone to errors: Is it easy to make mistakes when doing the task manually?
Data entry, report generation, and sending regular emails are prime examples of tasks that can be automated with Apps Script.
Getting Started with Google Apps Script
Google Apps Script is based on JavaScript and lets you add functionality to Google Workspace apps like Sheets, Docs, and Gmail. If you know JavaScript, you'll feel right at home. Even if you don't, the basics are pretty easy to pick up.
A core concept is using Apps Script's APIs to interact with Google services. For example, here's how to read data from a Google Sheet:
function readData() {
const sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
const data = sheet.getDataRange().getValues();
Logger.log(data);
}
This simple script grabs all the data from the current sheet and logs it. A great starting point!
Automating Data Collection with Web Scraping
Web scraping is a powerful way to automatically collect data from websites. Let's say you need to pull daily news headlines. Here's how Apps Script can help:
function scrapeHeadlines() {
var url = 'https://www.bbc.com/'
var response = UrlFetchApp.fetch(url)
var html = response.getContentText()
// Load the HTML into HtmlService for parsing
var document = HtmlService.createHtmlOutput(html).getContent()
// Extract headlines using regular expressions or a combination of string manipulations
var headlines = []
var regex = /<h2[^>]*data-testid="card-headline"[^>]*>(.*?)<\/h2>/g
var match
while ((match = regex.exec(document))) {
// Clean up the matched headline text
var headline = match[1].replace(/<!--.*?-->/g, '').trim()
headlines.push(headline)
}
Logger.log(headlines)
return headlines; // Return the headlines
}
function storeHeadlines() {
var headlines = scrapeHeadlines() // Assume this function returns an array of headlines
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet()
for (var i = 0; i < headlines.length; i++) {
sheet.appendRow([headlines[i]])
}
}
This script fetches the HTML content of the webpage and uses a regular expression to extract the headlines. The UrlFetchApp service allows you to make HTTP requests, while the regex helps in parsing the HTML. It takes the headlines array and appends each headline as a new row in the active sheet. Now, you have a dynamic and up-to-date list of news headlines without lifting a finger.
Automating Email Notifications
Sending emails manually can be a real chore. Apps Script can automate this process. Here's a basic example:
function sendEmail(recipient, subject, body) {
MailApp.sendEmail(recipient, subject, body);
}
function sendPersonalizedEmails() {
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("Contacts"); // Specify sheet name
const data = sheet.getDataRange().getValues();
for (let i = 1; i < data.length; i++) { // Start from row 2 to skip headers
const name = data[i][0];
const email = data[i][1];
const subject = `Hello ${name}`;
const body = `Dear ${name},\n\nThis is a personalized email.`;
sendEmail(email, subject, body)
}
}
This script loops through the data, crafting and sending a personalized email to each recipient. It’s a simple yet effective way to ensure everyone gets the information they need.
Automating Report Generation
Apps Script makes it easy to create automated reports. The process involves creating a template in Google Sheets and then using a script to populate it with data:
function generateSalesReport() {
const spreadsheet = SpreadsheetApp.getActiveSpreadsheet();
const sheet = spreadsheet.getSheetByName("SalesReport"); // Get the destination sheet by name
const template = SpreadsheetApp.openById('templateId').getSheetByName('Template');
const data = getSalesData(); // Your function to fetch sales data
// Clear the destination sheet before copying the template
sheet.clearContents();
template.copyTo(spreadsheet).setName("SalesReportCopy"); // Copy the template
const copiedSheet = spreadsheet.getSheetByName("SalesReportCopy");
copiedSheet.getRange('B2').setValue('Sales Report for ' + new Date());
// Append data starting from the next available row
const lastRow = sheet.getLastRow();
const startRow = lastRow + 1;
for (let i = 0; i < data.length; i++) {
sheet.appendRow([data[i].date, data[i].amount, data[i].category]);
}
// Optionally, delete the copied sheet if you don't need it
spreadsheet.deleteSheet(copiedSheet);
}
This script copies the template to a new sheet, sets the report title, and populates it with sales data. You can extend this script to include charts, summaries, and more.
Integrating with External APIs
One of the most powerful features of Apps Script is its ability to integrate with external APIs. This allows you to bring in data from various sources and create a seamless workflow.
Here’s an example using a weather API:
function fetchWeatherData(location) { // Accepts location as a parameter
const apiKey = 'your_api_key'; // Store API keys securely, e.g., in Script Properties
const url = `https://api.weatherapi.com/v1/current.json?key=${apiKey}&q=${location}`;
const response = UrlFetchApp.fetch(url);
const data = JSON.parse(response.getContentText());
return data;
}
function generateSalesReportWithWeather(location = 'London') { // Default location
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet()
var template = SpreadsheetApp.openById('templateId').getSheetByName('Template')
var data = getSalesData() // Assume this function fetches sales data
const weather = fetchWeatherData(location); // Pass location to fetchWeatherData
template.copyTo(sheet)
sheet.getRange('B2').setValue('Sales Report for ' + new Date())
sheet.getRange('B4').setValue(
`Current Weather in ${location}: ${weather.current.condition.text}, ${weather.current.temp_c}°C`
);
for (var i = 0; i < data.length; i++) {
sheet.appendRow([data[i].date, data[i].amount, data[i].category])
}
}
This script adds a line to include the current weather in the sales report, providing context alongside the sales data. This can be adapted based on your use-case and requirements.
Real-time Updates with Triggers
Triggers automate scripts based on events. They can automate tasks like sending emails or updating data in response to changes. For example, you could trigger a script to run every hour or when a form is submitted.
function sendEmailOnFormSubmit(e) {
var name = e.values[0]
var email = e.values[1]
var subject = 'New Form Submission'
var body = 'Name: ' + name + '\nEmail: ' + email
MailApp.sendEmail(email, subject, body)
}
function createFormSubmitTrigger() {
// Use a specific form ID
const form = FormApp.openById('your_form_id');
ScriptApp.newTrigger('sendEmailOnFormSubmit')
.forForm(form)
.onFormSubmit()
.create();
}
This adds the crucial step of actually creating the trigger. Remember to replace 'your_form_id'
with the actual ID of your form.
Using Webhooks for Real-time Data
Webhooks allow you to receive real-time data from external services. For example, you can set up a webhook to receive notifications from a CRM system.
function doPost(e) {
try {
const data = JSON.parse(e.postData.contents);
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("WebhookData"); // Use a specific sheet
sheet.appendRow([data.name, data.email, data.message]);
return ContentService.createTextOutput(JSON.stringify({ status: 'success' })).setMimeType(ContentService.MimeType.JSON);
} catch (error) {
Logger.log(`Error processing webhook: ${error}`);
return ContentService.createTextOutput(JSON.stringify({ status: 'error', message: error.message })).setMimeType(ContentService.MimeType.JSON).setStatusCode(500);
}
}
This script handles incoming POST requests, parses the data, and appends it to a Google Sheet. You can set up the webhook in your CRM system to send data to this endpoint.
Conclusion: Unlock the Power of Automation
Google Apps Script is a game-changer for anyone working with data in Google Workspace. Start small, focus on automating your most tedious tasks, and watch your productivity soar. I hope this guide has given you a good starting point. Happy automating!