- Published on
The Future of Reporting is Visual - Automate, Integrate, and Elevate with Slack & Google Cloud
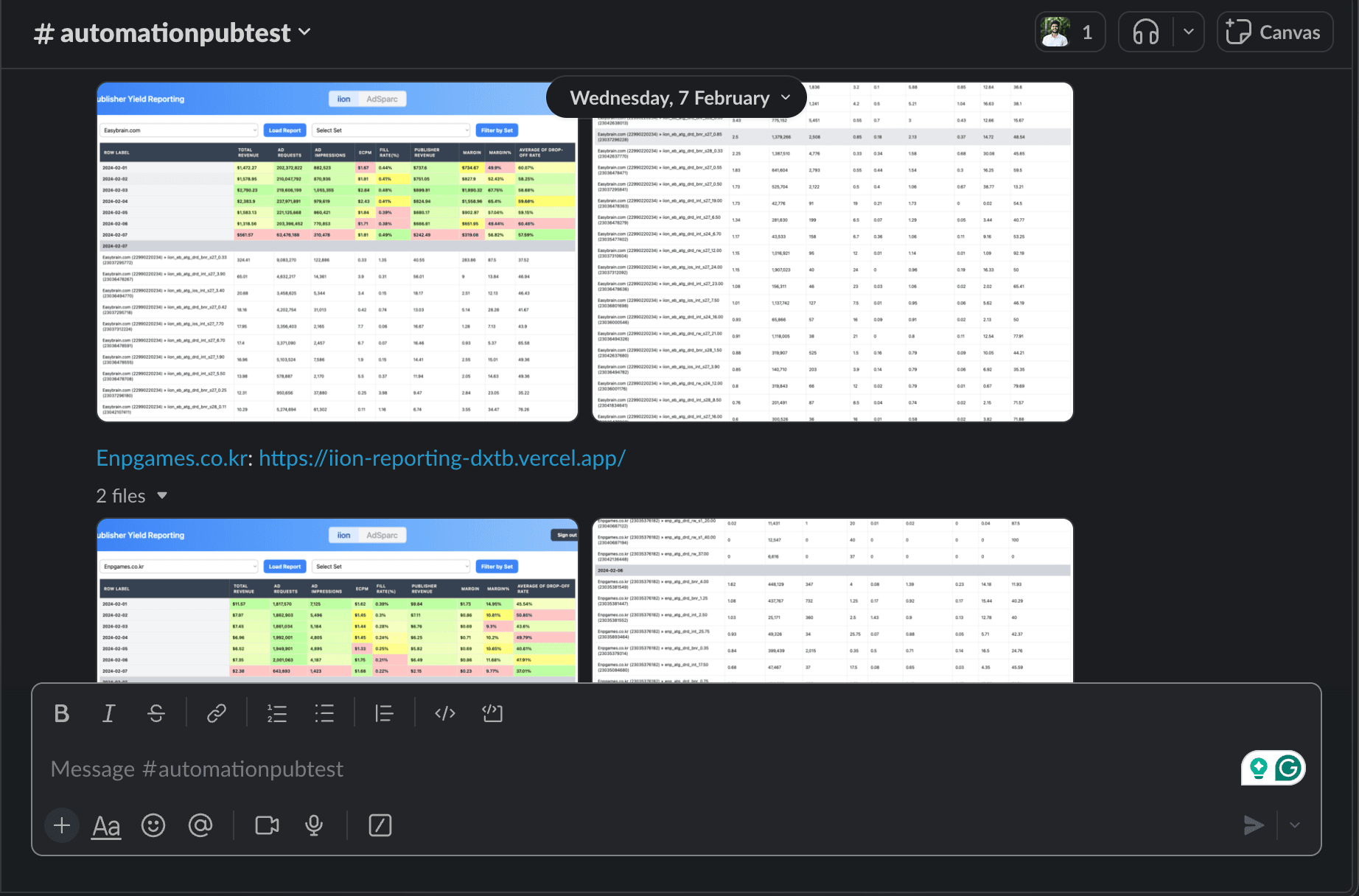
Enhance team communication and data-driven decision-making with automated visual reporting. This blog post explores how to leverage Google Cloud Functions and Puppeteer to capture website screenshots and seamlessly share them on Slack. Explore the benefits of this powerful automation for improved collaboration and insights.
1. Integrating Puppeteer for Screenshot Capture
What is Puppeteer?
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium browsers. It's commonly used for automated testing and web scraping. In our scenario, Puppeteer will navigate to specific URLs and capture screenshots.
Setting Up Puppeteer
Add Puppeteer to your project by adding a .puppeteerrc.cjs file:
const { join } = require('path')
/**
* @type {import("puppeteer").Configuration}
*/
module.exports = {
// Changes the cache location for Puppeteer.
cacheDirectory: join(__dirname, '.cache', 'puppeteer'),
}
Then, create a function to capture screenshots. Here's a snippet:
const { launch } = require('puppeteer')
async function captureScreenshot(page) {
try {
const screenshotBuffer = await page.screenshot({
encoding: 'binary',
clip: {
x: 250,
y: 400,
width: 1500,
height: 900,
},
})
return [screenshotBuffer]
} catch (error) {
console.error('Error capturing screenshot:', error.message)
throw error
}
}
This function captures a screenshot of the specified area on the webpage.
2. Sending Screenshots to Slack
Introduction to Slack API
Slack provides a robust API for integrating custom applications. We'll use Slack's Web API to upload screenshots to specific channels. First, you need to create a Slack app and generate an access token.
Setting Up Slack Integration
Create a function to send screenshots to Slack:
const { WebClient } = require('@slack/web-api')
async function sendReportsOnSlack(screenshotBuffers, channelId, initialComment) {
const slackAccessToken = process.env.SLACK_ACCESS_TOKEN
const slackClient = new WebClient(slackAccessToken)
try {
await slackClient.files.uploadV2({
file_uploads: [{ file: screenshotBuffers[0], filename: 'screenshot.png' }],
channel_id: channelId,
initial_comment: initialComment,
})
console.log('Report posted to Slack')
} catch (error) {
console.error('Error sending report to Slack:', error.message)
throw error
}
}
This function uploads the screenshot to the specified Slack channel.
3. Combining Everything in a Cloud Function
Full Function Code
Integrate the screenshot capture and Slack upload functions into a single Cloud Function:
const functions = require('@google-cloud/functions-framework')
const { launch } = require('puppeteer')
const { WebClient } = require('@slack/web-api')
const defaultChannelId = 'C06FATQBZ0D'
functions.http('captureAndSendScreenshot', async (req, res) => {
try {
const browser = await launch({ headless: true })
const page = await browser.newPage()
await page.setViewport({ width: 1920, height: 1300 })
const urls = [
{ url: 'https://example.com/report1', name: 'Report 1' },
{ url: 'https://example.com/report2', name: 'Report 2' },
]
for (const { url, name } of urls) {
await page.goto(url)
await page.waitForTimeout(15000)
const screenshotBuffers = await captureScreenshot(page)
await sendReportsOnSlack(screenshotBuffers, defaultChannelId, name)
}
await browser.close()
res.status(200).send('Screenshots captured and sent to Slack')
} catch (error) {
console.error('Error:', error)
res.status(500).send('An error occurred')
}
})
Deploy this function using the gcloud
command.
4. Real-World Use Case: Automated Report Sharing
Scenario: Marketing Team
Challenge: Marketing teams rely on daily performance dashboards to track campaign effectiveness. However, manually capturing screenshots and distributing them across the team is time-consuming and inefficient, potentially leading to delays in identifying issues or opportunities.
Solution: Implement automated visual reporting to deliver up-to-date screenshots of key marketing dashboards directly to the team's Slack channel each morning. This provides immediate access to critical data, facilitating quicker analysis, data-driven discussions, and faster decision-making.
Scenario: Development Team
Challenge: Development teams need to closely monitor the status of various deployments and environments to ensure system stability and uptime. Constantly checking multiple dashboards and manually sharing updates is inefficient and prone to human error.
Solution: Utilize automated screenshots of monitoring dashboards delivered directly to the development team's Slack channel. This ensures real-time visibility into system health, enabling the team to promptly identify and address any anomalies or performance issues without manual effort or potential oversights.
Scenario: Publisher Success Team
Challenge: Publisher success teams need to monitor the performance of ad campaigns across various platforms for their clients. This involves tracking metrics like impressions, clicks, and conversions, often requiring manual report pulling and sharing.
Solution: By automating visual reports, the team can receive daily or hourly screenshots of key campaign dashboards directly in their Slack channel. This provides a constant pulse on campaign health, enabling quicker optimization decisions and proactive communication with clients.
Scenario: E-commerce Operations
Challenge: E-commerce operations teams require constant visibility into website performance, inventory levels, and order fulfillment. Manually checking various dashboards is inefficient and prone to delays.
Solution: Automated screenshots of key dashboards – website traffic, top-selling products, out-of-stock alerts, order fulfillment progress – can be sent to the team's Slack channel at regular intervals. This provides a real-time snapshot of operations, facilitating proactive issue identification and faster response times.
Scenario: Executive Team
Challenge: Executives need a high-level overview of business performance but may not have time to delve into individual reports.
Solution: Curated visual dashboards summarizing key metrics like revenue, customer acquisition cost, and website traffic can be automatically captured and shared in a dedicated Slack channel. This allows executives to quickly grasp performance trends and make informed decisions.
Scenario: Sales Team
Challenge: Sales teams can benefit from timely insights into lead generation campaigns and pipeline health to prioritize efforts effectively.
Solution: Automated screenshots of dashboards displaying lead generation sources, conversion rates, and sales pipeline stages can be delivered to the sales team's Slack channel. This enables them to track campaign effectiveness, identify potential bottlenecks, and focus on high-priority opportunities.
Key Takeaway: These examples demonstrate the wide applicability of automated visual reporting across various teams and industries. By leveraging tools like Google Cloud Functions, Puppeteer, and Slack, businesses can streamline communication, improve data visibility, and empower teams to make better, faster decisions.
5. Handling Errors and Exceptions
Common Errors
Errors such as network issues or authentication failures can disrupt the automation process. It's essential to handle these gracefully and provide meaningful error messages.
Implementing Error Handling
Enhance your functions with try-catch blocks and meaningful log messages:
async function captureScreenshot(page) {
try {
const screenshotBuffer = await page.screenshot({ encoding: 'binary' })
return [screenshotBuffer]
} catch (error) {
console.error('Error capturing screenshot:', error.message)
throw error
}
}
async function sendReportsOnSlack(screenshotBuffers, channelId, initialComment) {
try {
const slackAccessToken = process.env.SLACK_ACCESS_TOKEN
const slackClient = new WebClient(slackAccessToken)
await slackClient.files.uploadV2({
file_uploads: [{ file: screenshotBuffers[0], filename: 'screenshot.png' }],
channel_id: channelId,
initial_comment: initialComment,
})
console.log('Report posted to Slack')
} catch (error) {
console.error('Error sending report to Slack:', error.message)
throw error
}
}
6. Optimizing the Workflow
Improving Performance
Ensure the workflow is optimized by reducing unnecessary delays and improving the efficiency of Puppeteer operations. Use caching and avoid redundant operations where possible.
Security Considerations
Ensure that sensitive data such as API tokens are securely stored and accessed. Use environment variables and avoid hardcoding credentials in your source code.
Conclusion
Automating visual reporting using Google Cloud Functions and Puppeteer significantly enhances business efficiency and employee productivity. By following the detailed process outlined in this blog post, you can implement a robust solution that streamlines your reporting workflow and ensures timely, accurate data dissemination. Embrace the power of automation to unlock new levels of productivity and insight for your organization. Happy Automating!